Export Svelte as a component
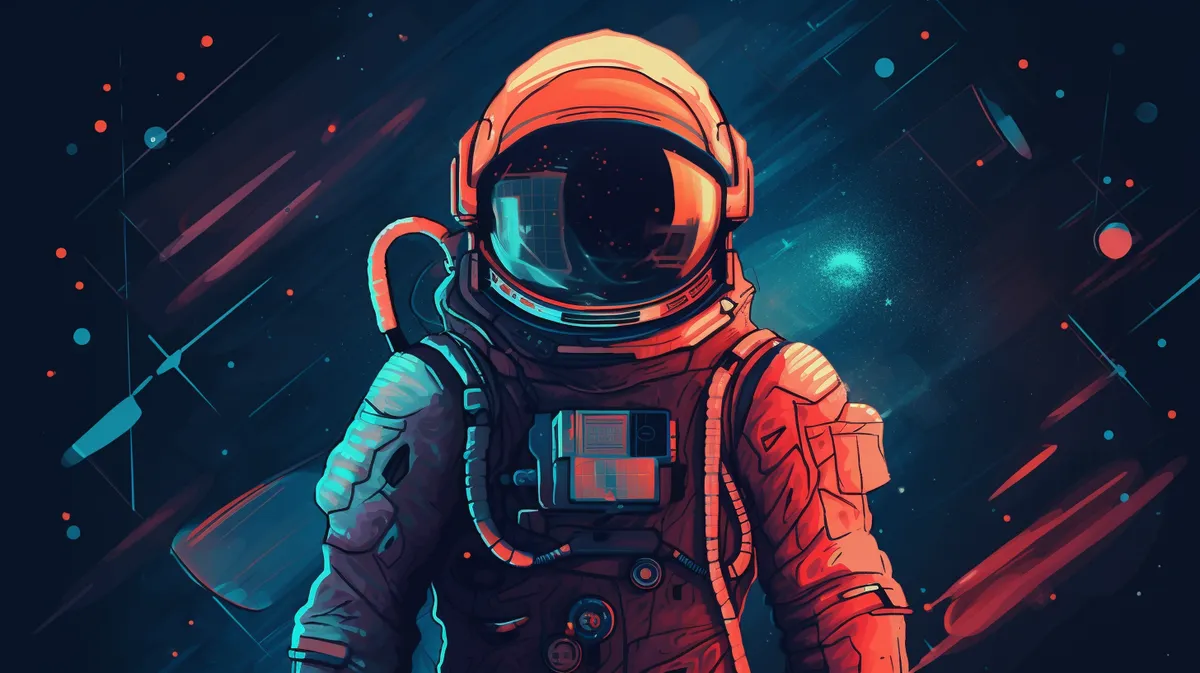
- svelte
- astroJS
Find out how to export your Svelte app as a component.
Integrating a Svelte Component into an Astro.js Project
Set Up a Svelte Project
- Initialize a new Svelte project:
npx degit sveltejs/template svelte-app cd svelte-app npm install
Create Your Svelte Component
- In
src
, create your Svelte component, e.g.,MyComponent.svelte
:<script> export let name; </script> <style> /* Add your styles here */ </style> <div> Hello {name}! </div>
Build the Svelte Component
- Install Vite and create a build configuration:
npm install vite @sveltejs/vite-plugin-svelte --save-dev
- Create a
vite.config.js
file in the root of your project:import { defineConfig } from 'vite'; import { svelte } from '@sveltejs/vite-plugin-svelte'; export default defineConfig({ plugins: [svelte()], build: { lib: { entry: 'src/MyComponent.svelte', name: 'MyComponent', fileName: (format) => `my-component.${format}.js` }, rollupOptions: { external: ['svelte/internal'], output: { globals: { 'svelte/internal': 'svelte' } } } } });
Build the Library
- Run the build command:
npx vite build
Include in Astro Project
-
Copy the built file (
dist/my-component.umd.js
) to your Astro project. -
In your Astro project, install the Svelte integration:
npm install @astrojs/svelte
-
Update
astro.config.mjs
to include the Svelte integration:import { defineConfig } from 'astro/config'; import svelte from '@astrojs/svelte'; export default defineConfig({ integrations: [svelte()], });
Use the Svelte Component in Astro
- In an Astro component, import and use the Svelte component:
--- import { SvelteComponent } from 'svelte'; import MyComponent from './path/to/my-component.umd.js'; --- <MyComponent name="Astro"/>