Adding Tailwind to Svelte
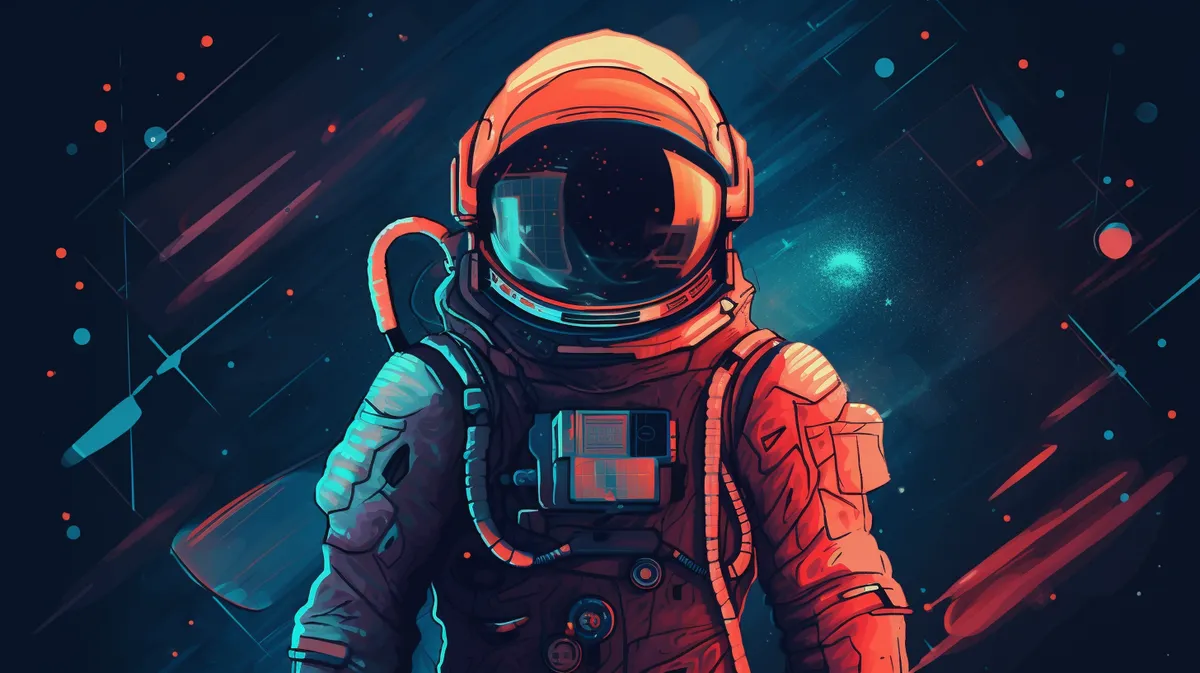
- svelte
- tailwind
Simple steps to add Tailwind to your Svelte App.
Adding Tailwind CSS to a Svelte Project
Install Tailwind CSS
-
Install Tailwind CSS and its dependencies:
npm install -D tailwindcss postcss autoprefixer
-
Create Tailwind and PostCSS configuration files:
npx tailwindcss init tailwind.config.cjs -p
Configure Tailwind CSS
-
Update
tailwind.config.cjs
to specify the paths to all of your template files:/** @type {import('tailwindcss').Config} */ module.exports = { content: [ './src/**/*.{html,js,svelte,ts}' ], theme: { extend: {}, }, plugins: [], };
-
Add the Tailwind directives to your CSS:
- Create a
src/global.css
file and add the following:@tailwind base; @tailwind components; @tailwind utilities;
- Create a
Include Tailwind CSS in Your Project
- Update your
src/main.js
to import the global CSS file:import './global.css'; import App from './App.svelte'; const app = new App({ target: document.body, props: { name: 'world' } }); export default app;
Use Tailwind CSS in Svelte Components
- You can now use Tailwind CSS classes in your Svelte components:
<script> export let name; </script> <style> /* Add component-specific styles here */ </style> <div class="p-4 bg-blue-500 text-white rounded"> Hello {name}! </div>
Build and Run the Project
- Start the development server to see your changes:
npm run dev